When running the terraform init command it will store the current state locally in files. This is pretty convenient but if that file is stored on your workstation that makes it impossible to share the build process between multiple developers. This would also make it impossible to add this to a CI/CD pipeline if the actual state was dependent on a specific pc.
Clever scripts could be done to save this information on a networked drive but why bother when this same information could be saved within AWS itself. With a small bit of configuration it is possible to save the infrastructure state in a S3 bucket. To ensure that only one person can work on the infrastructure at a time the AWS dynamo database would be used to store the locking state.
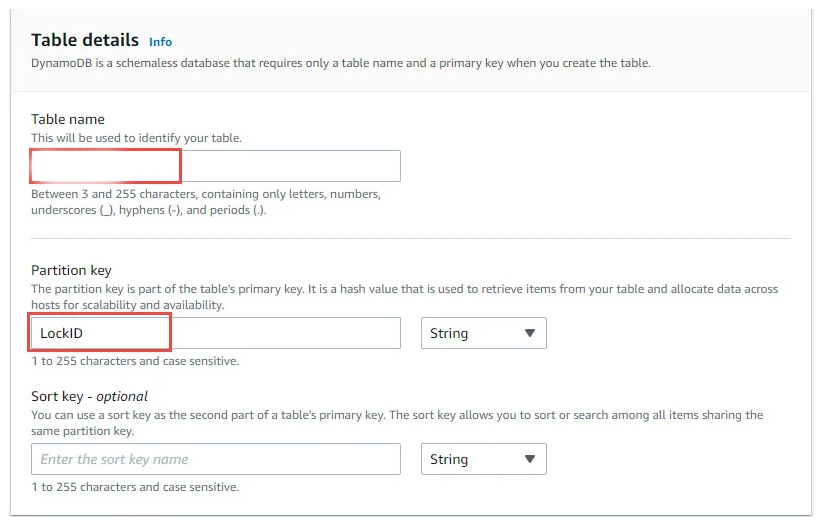
Once the dynamodb table created with the Partition key equal to “LockID” and with the S3 bucket created all the AWS backend setup is complete. Simply refer to this table and this bucket in your configuration.
main.tf |
provider “aws” { region = var.region access_key = var.AWS_ACCESS_KEY_ID secret_key = var.AWS_SECRET_ACCESS_KEY } terraform { backend “s3” { bucket = “cgd.development.terraform.state” key = “terraform.tfstate” region = “us-east-1” dynamodb_table = “cgd-development-dynamodb-table” } } |
However, it does not matter if the Terraform state is saved locally or saved in S3 the first step still needs to be the initialization with the “terraform init” command.
Initializing the backend… Successfully configured the backend “s3”! Terraform will automatically use this backend unless the backend configuration changes. Initializing provider plugins… – Finding latest version of hashicorp/aws… – Installing hashicorp/aws v4.20.0… – Installed hashicorp/aws v4.20.0 (signed by HashiCorp) Terraform has created a lock file .terraform.lock.hcl to record the provider selections it made above. Include this file in your version control repository so that Terraform can guarantee to make the same selections by default when you run “terraform init” in the future. Terraform has been successfully initialized! You may now begin working with Terraform. Try running “terraform plan” to see any changes that are required for your infrastructure. All Terraform commands should now work. If you ever set or change modules or backend configuration for Terraform, rerun this command to reinitialize your working directory. If you forget, other commands will detect it and remind you to do so if necessary. |
The output from the initialization in this scenario is quite similar to the local case but taking a careful look we can see that the S3 bucket is being used.
The configuration is identical the previous blog with the exception of the backend configuration and the changes to the output.
output.tf |
output “region” { value = var.region } output “_environment” { value = var.environment } output “awskey” { value = “don’t display this” } output “awssecret” { value = “don’t display this” } |
This configuration for Terraform still doesn’t create any AWS infrastructure but it is the perfect starting point to be included into a CI/CD pipeline.